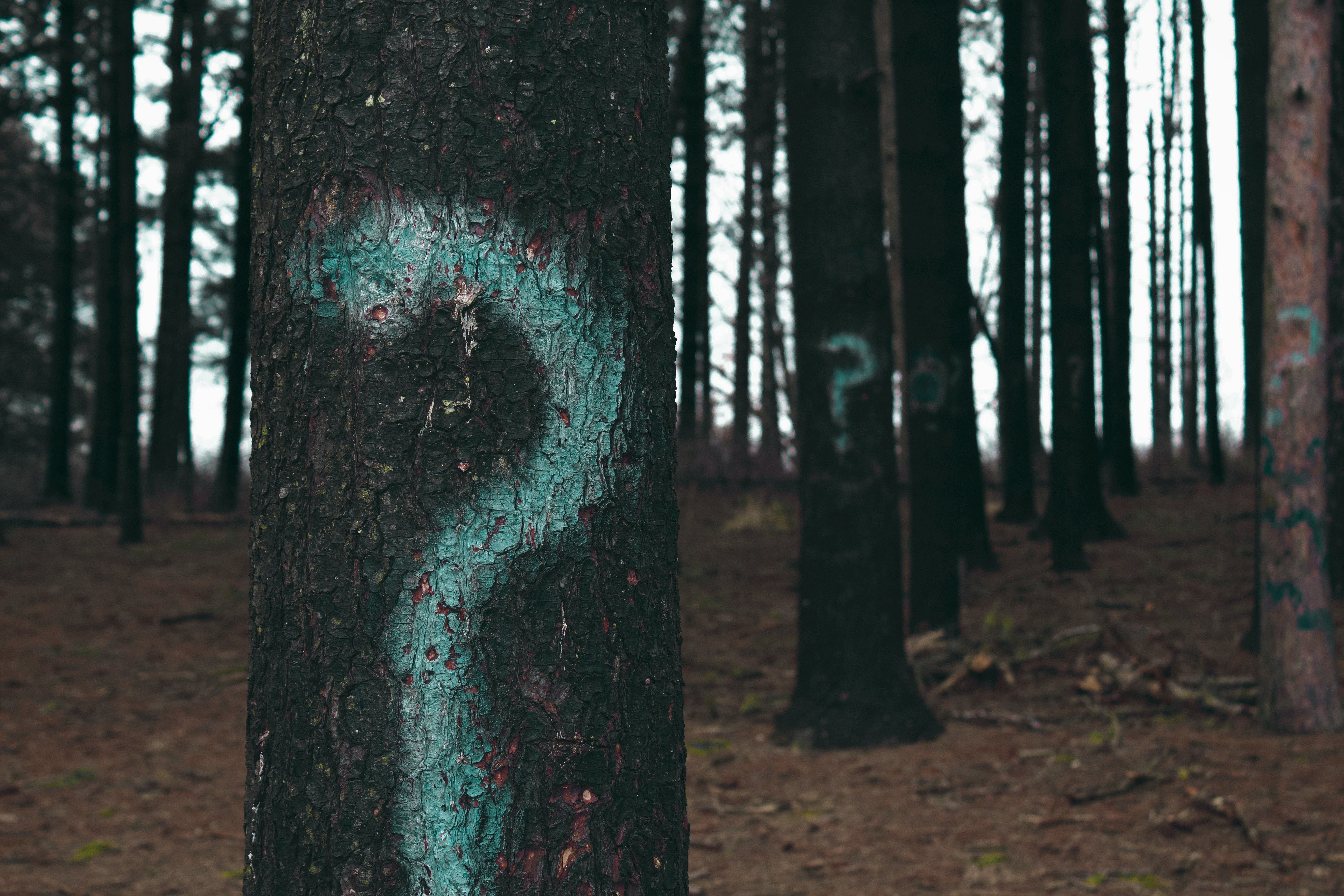
Blockchain Job Interview Warm‑Up: 30 Real Coding & System‑Design Questions
Blockchain technology has evolved from a niche concept powering cryptocurrency to a full-fledged framework for decentralised applications across multiple industries. From smart contracts and decentralised finance (DeFi) to supply chain management and digital identity systems, blockchain is reinventing how we transact and interact in the digital world. As a result, blockchain developers, architects, and entrepreneurs are in high demand.
If you’re aiming to secure a blockchain‑related role—whether at a cutting-edge start-up, a multinational tech firm, or a forward‑thinking consultancy—chances are you’ll face a rigorous, multifaceted interview process. Beyond classic coding tasks, recruiters often delve into distributed systems, cryptographic principles, consensus algorithms, and smart contract security to evaluate your technical prowess and problem-solving skills.
In this article, we’ll explore 30 real coding & system-design questions you might encounter on your blockchain job interview. We’ll also discuss why proper preparation is crucial, and how you can stand out from the competition by showcasing both your technical and conceptual understanding.
For a curated selection of the latest blockchain job vacancies, head over to www.blockchainjobs.uk. There, you’ll find roles across the UK that span development, architecture, project management, and more. Let’s dive right in so you can approach your next blockchain interview with confidence.
1. Why Blockchain Interview Preparation Matters
The blockchain space is still rapidly evolving. New frameworks, consensus protocols, and cryptographic techniques emerge at a pace that can feel dizzying. However, one constant remains: employers want blockchain specialists who can apply robust programming skills and design scalable, secure systems that align with real-world requirements.
Here are some core reasons why structured interview prep is crucial in the blockchain sector:
Demonstrate Technical Depth
Blockchain relies on principles from cryptography, distributed computing, network theory, and game theory. Your interviewers will test whether you can integrate these concepts effectively.
Demonstrating hands-on programming experience with languages like Solidity, Go, Rust, or even TypeScript for smart contract development or chain tooling is essential.
Prove Your Security Awareness
Blockchain solutions handle valuable data or digital assets, making them frequent targets of hacking attempts.
Employers want to see that you’ve considered attack vectors, secure coding practices, and auditing measures for on-chain and off-chain components.
Showcase System Design Competence
It’s not just about writing a smart contract. It’s also about how you architect entire ecosystems: from user authentication and data storage to consensus mechanisms and chain interoperability.
Employers value professionals who can design robust, cost-effective, and low-latency solutions in a decentralised environment.
Navigate Regulatory & Ethical Considerations
In the UK and beyond, regulatory frameworks for digital assets and blockchain services are evolving.
Companies want employees who have at least a basic grasp of compliance (such as FCA guidelines) and who understand the ethical implications of decentralised technologies.
Validate Communication Skills
Blockchain initiatives often involve cross-functional collaboration among developers, business teams, and external stakeholders.
Strong communication—especially the ability to explain complex protocols to non-technical team members—sets top candidates apart.
By dedicating time to comprehensive interview preparation, you’ll be better positioned to handle both the technical and conceptual questions that come your way. Let’s start our deep dive with the coding segment.
2. 15 Real Coding Interview Questions
While blockchain interviews often focus on system-level thinking, you’ll still need to prove your coding mettle. Below are 15 coding questions that recruiters across the industry frequently ask. Think of these as opportunities to show your algorithmic skills, your domain knowledge of blockchain-specific data structures, and your practical coding abilities.
Coding Question 1: Implement a Merkle Tree
Question: Implement a basic Merkle Tree data structure, including the ability to generate a Merkle Root and verify membership for a given leaf.
What to focus on:
Hashing strategy (e.g., SHA-256).
Handling odd number of leaf nodes.
Generating proofs of inclusion.
Coding Question 2: Chain Reorganisation
Question: Write a function that takes two blockchain branches (each a linked structure of blocks) and determines which one is valid and longer, simulating a blockchain chain reorganisation event.
What to focus on:
Understanding of longest chain rule or heaviest chain rule.
Properly handling potential forks and reorganisations.
Data structure manipulations (linked lists, pointers, etc.).
Coding Question 3: Basic Wallet Functionality
Question: Given an address and a private key, create a function that signs a transaction and returns a valid transaction object.
What to focus on:
Basic knowledge of public‑private key cryptography (ECDSA).
Understanding of transaction formats (inputs, outputs).
Handling hashing steps prior to signing.
Coding Question 4: Gas Calculation in Smart Contracts
Question: If you have a smart contract function with multiple SSTORE operations, implement a function that calculates the gas cost given a set of operations and current states.
What to focus on:
EVM opcodes and cost rules (especially for Ethereum).
Distinguishing between storage overwrite and new storage.
Potential edge cases in cost refunds.
Coding Question 5: Convert an Integer to a Solid-Byte Hex
Question: Write a function that converts an integer to its big-endian hex representation, suitable for smart contract or blockchain data encoding.
What to focus on:
Byte manipulation in languages like C++, Python, or Go.
Endianness awareness.
Format consistency for off-chain/on-chain communication.
Coding Question 6: Implement a Simple Proof of Work
Question: Create a function that, given a block header and a target difficulty, tries different nonces until it finds a valid hash (below the target).
What to focus on:
Efficient hashing loops (avoid performance overheads).
Understanding of the target–difficulty relationship in PoW systems.
Handling nonce overflow and re-initialisation.
Coding Question 7: Secure Hash with Salt
Question: Write a function that stores user credentials by hashing the password with a random salt, ensuring secure storage.
What to focus on:
Best practices in cryptographic hashing (e.g., scrypt, Argon2, or at least PBKDF2).
Generating a random salt securely.
Properly combining salt and password pre-hashing.
Coding Question 8: Deterministic Key Derivation
Question: Implement a function that derives child keys from a master key following the BIP32/BIP44 hierarchical deterministic wallet standard.
What to focus on:
HMAC-SHA512 steps for child key derivation.
Handling hardened vs. non-hardened derivations.
Input formats and extended keys (xPub, xPriv).
Coding Question 9: Deploy & Test a Simple Smart Contract
Question: Demonstrate how you’d write, deploy, and test a “Hello World” smart contract on Ethereum using a scripting framework (e.g., Hardhat, Truffle).
What to focus on:
Solidity basics, contract structure.
Deployment scripts (JavaScript/TypeScript).
Writing and running unit tests (Mocha/Chai or similar).
Coding Question 10: Parsing a Blockchain Block Header
Question: Parse a block header from a hex string, extracting fields such as version, previous block hash, merkle root, timestamp, bits (target), and nonce.
What to focus on:
Byte offsets and endianness.
Converting hex to numeric fields.
Real-world relevance to Bitcoin or similar blockchains.
Coding Question 11: Implement a Simple KV Store Smart Contract
Question: Write a contract that stores a key-value mapping in persistent storage, with setter and getter functions.
What to focus on:
Proper use of mapping in Solidity.
Gas considerations when storing/updating data.
Basic access control checks (e.g., only owner can modify).
Coding Question 12: NFT Minting Function
Question: Implement a function that mints a new ERC-721 token to a specified address with a unique token URI.
What to focus on:
Understanding of ERC-721 standard methods (e.g.,
_safeMint
,_setTokenURI
).Potential re-entrancy or front-running concerns.
Maintaining a robust metadata registry.
Coding Question 13: Checkpointing Mechanism
Question: Given a list of block hashes, implement a checkpoint system that ensures the chain is validated up to certain known “safe” points.
What to focus on:
Verifying hash consistency and block height.
Handling forks past the checkpoint.
Designing for fast sync or light client usage.
Coding Question 14: Off-Chain Data Signing and Verification
Question: Write two functions—one to sign arbitrary data off-chain (via private key) and another to verify that signature on-chain in a smart contract.
What to focus on:
ECDSA signature structure (r, s, v).
eth_sign vs. EIP-712 typed data.
Handling potential signature malleability issues.
Coding Question 15: Zero-Knowledge Proof Integration
Question: Show how you’d integrate a basic zk-SNARK verification (e.g., Groth16) in a smart contract environment (pseudocode is fine).
What to focus on:
High-level steps of generating proofs off-chain.
On-chain verification contract usage.
Gas optimisations for cryptographic proofs.
When tackling these coding problems, focus on:
Efficiency and reliability: Blockchain networks can be resource-constrained.
Security best practices: Minimising vulnerabilities.
Readability: Interviewers appreciate code that’s clear and logically structured.
3. 15 System & Architecture Design Questions
Blockchain interviews often dedicate a significant portion to system design—evaluating how well you can conceptualise and build scalable, secure, decentralised infrastructures. Below are 15 questions focusing on architecture, consensus mechanisms, on-chain/off-chain interactions, and more. Understanding these aspects is vital for designing robust blockchain solutions.
System Design Question 1: Designing a Permissioned Blockchain
Scenario: You must design a blockchain for an enterprise consortium, allowing only authorised nodes to participate.
Key Points to Discuss:
Consensus algorithms (PBFT, Raft, IBFT).
Identity management (certificates, MSP in Hyperledger Fabric).
Governance model (admin, endorsing peers, membership revocation).
System Design Question 2: Layer 2 Scaling Solution
Scenario: Build a Layer 2 network for faster transactions without altering the main chain’s consensus.
Key Points to Discuss:
Payment channels (e.g., Lightning Network).
State channels or rollups (Optimistic, ZK-Rollups).
Trade-offs: throughput vs. security vs. complexity.
System Design Question 3: Multi-Chain Interoperability
Scenario: You want to enable asset transfer between Ethereum and a custom sidechain.
Key Points to Discuss:
Bridging techniques (lock-and-mint, burn-and-redeem).
Security concerns around custodial or non-custodial bridges.
Handling chain reorgs and finality.
System Design Question 4: NFT Marketplace Architecture
Scenario: Develop a decentralised marketplace for ERC-721 tokens.
Key Points to Discuss:
Smart contract design (listing, bidding, escrow).
User experience with wallets (MetaMask, WalletConnect).
Off-chain metadata storage (IPFS, Arweave) vs. on-chain data.
System Design Question 5: On-Chain Governance Module
Scenario: You’re building a DAO (Decentralised Autonomous Organisation) module for protocol upgrades.
Key Points to Discuss:
Voting mechanisms (token-weighted, quadratic voting).
Proposal lifecycle (submission, discussion, voting, execution).
Guarding against vote manipulation or whale concentration.
System Design Question 6: Identity & KYC on the Blockchain
Scenario: Integrate a decentralised identity (DID) solution to comply with KYC regulations for a financial DApp.
Key Points to Discuss:
DID standards (W3C), Verifiable Credentials.
Privacy-preserving techniques (ZKPs for identity proofs).
Mapping on-chain addresses to verified identities without exposing personal data.
System Design Question 7: Tokenising Real-World Assets
Scenario: Tokenise real-estate assets on a public blockchain for fractional ownership.
Key Points to Discuss:
Smart contract representation (ERC-20, ERC-721, or specialised standard).
Regulatory compliance in the UK or abroad (securities laws).
Management of off-chain legal documents (proof of ownership).
System Design Question 8: Hybrid Centralised/Decentralised Architecture
Scenario: You have an enterprise solution where certain data must stay private, while key transactions must be on a blockchain.
Key Points to Discuss:
Off-chain data storage (SQL, IPFS, private data vaults).
Access control layers (private vs. public sub-chains).
Synchronising events between centralised databases and blockchain ledgers.
System Design Question 9: Oracle Integration for Real-World Data
Scenario: Your smart contracts need live price data for commodities.
Key Points to Discuss:
Oracle frameworks (Chainlink, Band Protocol).
Avoiding single point of failure.
Potential issues with data feed latency or manipulation (front-running).
System Design Question 10: Blockchain Analytics Platform
Scenario: Build a tool to analyse transaction patterns across multiple blockchains for anti-money laundering (AML) compliance.
Key Points to Discuss:
Data ingestion from different chains (Bitcoin, Ethereum, etc.).
Processing huge volumes of transactions (Big Data frameworks).
Visualisation and risk scoring (clustering addresses, identifying mixers).
System Design Question 11: Sharding for Scalability
Scenario: Propose a sharding mechanism to improve throughput on a high-traffic blockchain network.
Key Points to Discuss:
Network vs. state sharding.
Inter-shard communication protocols.
Potential concurrency issues or cross-shard atomicity.
System Design Question 12: Building a DeFi Lending Protocol
Scenario: Your team wants to launch a protocol allowing users to lend and borrow tokens.
Key Points to Discuss:
Liquidity pool design (interest rate models, e.g., Aave or Compound style).
Collateral management (margin calls, liquidation).
Risk assessment (impermanent loss, governance attacks).
System Design Question 13: Blockchain-Based Supply Chain Tracking
Scenario: Implement end-to-end tracking of goods, from raw materials to retail, using a blockchain ledger.
Key Points to Discuss:
IoT sensors or barcodes for data collection.
Smart contract logic for item provenance.
Data privacy (some data might be confidential to participants).
System Design Question 14: Consortium vs. Public Chain
Scenario: You’re tasked with deciding whether to use a public chain like Ethereum or a private consortium chain for a healthcare records system.
Key Points to Discuss:
Requirements for patient data privacy (HIPAA, GDPR).
Permissioning vs. trustless environment.
Transaction throughput and cost constraints.
System Design Question 15: Layer 1 Blockchain from Scratch
Scenario: You’ve been asked to design a new Layer 1 blockchain protocol focusing on fast finality and minimal environmental impact.
Key Points to Discuss:
Choice of consensus mechanism (PoS, DPoS, BFT variants).
Node software architecture (execution layer, networking, storage).
Monetary policy (token issuance, inflation, fees).
In these architecture questions, the interviewer is assessing:
Conceptual clarity: Do you understand distributed systems, consensus algorithms, and cryptographic fundamentals?
Trade-off analysis: Can you navigate the complexity of privacy vs. performance, or on-chain vs. off-chain data?
Practical feasibility: Are your designs implementable given real-world constraints such as regulations, budgets, and user adoption?
4. Tips for Conquering Blockchain Job Interviews
Acing a blockchain job interview goes beyond memorising coding patterns or parroting consensus definitions. Here are some proven strategies to help you showcase your skills and secure that coveted job offer.
Master the Fundamentals
Brush up on data structures (Merkle trees, Patricia tries), cryptographic primitives (ECDSA, hashing), and networking.
Understand how and why blockchains store transactions and ensure data integrity.
Build a Portfolio of Projects
Contribute to open-source blockchain repos or create personal projects (e.g., simple DeFi or NFT dApps).
Real-world code samples demonstrate practical expertise better than any CV description can.
Stay Current with Industry Developments
Keep up with major protocol updates (e.g., Ethereum’s transitions, Bitcoin Taproot).
Follow leading projects and standards (ERC-20, ERC-721, EIP-1559, etc.).
In the UK, watch how FCA regulations and government policy might shape crypto services.
Practice Smart Contract Security
Security is paramount—re-entrancy, integer overflow, access control are common pitfalls.
Familiarise yourself with tools like Slither or MythX for static analysis and auditing.
Get Comfortable with Whiteboard or Live Coding
Employers often test your logic in real time. Clarify your thinking process—talk aloud about trade-offs or potential pitfalls.
If you’re asked to code a snippet in Solidity or Go, ensure you can handle typical pitfalls (like array indices, data location in Solidity).
Explain Your Thought Process
Blockchain is complex. Interviewers want to see how you approach problems—break them down methodically, identifying security or scaling concerns.
Even if you don’t reach a final perfect solution, a structured approach can still impress.
Anticipate Behavioural Questions
Team culture matters. Expect queries about collaboration and conflict resolution.
Discuss how you’ve handled disagreements over design choices or feature prioritisation in previous roles or open-source projects.
Highlight Regulatory & Compliance Awareness
In the UK and worldwide, compliance is increasingly critical—especially in finance or healthcare.
Show basic knowledge of relevant frameworks (KYC/AML, GDPR for data privacy, potential stablecoin regulations).
Showcase Cross-Functional Collaboration
Blockchain projects often involve front-end devs, product managers, legal teams, and marketing.
Mention times you collaborated effectively, bridging technical and non-technical gaps.
Ask Smart Questions
End the interview by inquiring about long-term tech roadmaps, scalability visions, or how the company handles security audits.
This demonstrates genuine interest and a forward-thinking mindset.
Remember that blockchain is a broad domain. Tailor your answers to the specific role—be it core protocol development, dApp engineering, smart contract auditing, or enterprise blockchain solutions.
<a name="section5"></a>
5. Final Thoughts
The blockchain landscape represents a sea of opportunities—ranging from decentralised finance and gaming to supply chain and identity management. However, these opportunities come with high expectations regarding your ability to code efficiently, architect secure systems, and navigate a world where regulation and innovation collide daily.
By honing the 30 real coding & system-design questions discussed here, you’ll be better equipped to demonstrate practical expertise and conceptual depth during interviews. From building Merkle Trees and implementing basic Proof of Work, to designing cross-chain bridges and Layer 2 solutions, your interview readiness will shine through.
As you refine your knowledge and perfect your interview strategy, remember that this process is a two-way street. You also need to assess whether a prospective employer’s vision and work environment align with your professional goals. Ask about their technical stack, team culture, and approach to innovation.
If you’re looking for a new opportunity in the UK’s fast-growing blockchain ecosystem, www.blockchainjobs.uk is an excellent resource. There, you’ll find a variety of roles—ranging from protocol developers to solution architects—across diverse industries. Best of luck, and may your blockchain journey lead you to new heights of innovation and success!